React - First Steps
When it comes to web development, React is a big deal. It's a JavaScript library that helps you build cool and interactive user interfaces. The primary purpose is to split the application into small blocks of code, called components, like Lego pieces that can compound and nest each other. This approach allows developers to think ahead and reuse and customise those components for each case.
Some people love React and swear by it, while others think it's overhyped and not worth the effort. Nevertheless, it's clear that React has had a significant impact on modern web development, and it's not going away anytime soon. If you're looking to get into web development, you might want to start checking this blog post π
In this first entry, we will explore how React uses JavaScript native functions to hide specific details under the hood. It aims for a declarative implementation that makes the code more structured, easier to read (the code is often more read than modified) and reusable. This is the foundational concept on which React is built. After a little while, you will notice how wrapping an existing functionality to customise its behaviour and extend its capabilities is a common practice you will find daily when working in the industry or running your projects.
We will also explore how React creates new elements and positions them on the screen using the ReactDOM library.
And this is it! Welcome to this journey. I am glad to have you here to share the experience.
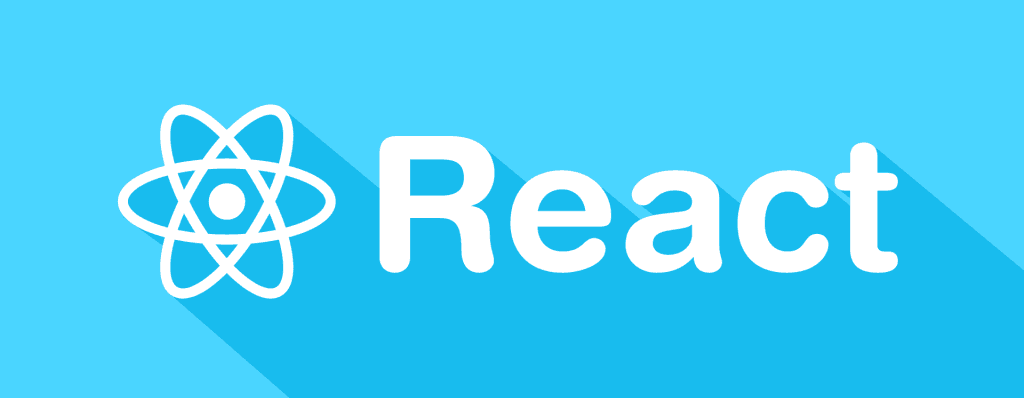
Why React?
#
React is like a gateway to modern web development because it's easy to start with and has tools familiar to JavaScript and jQuery users. You can transition your skills quickly if you come from the JS world. It is designed to build on existing practices rather than completely changing them.
React is more than just a familiar tool. It's a powerhouse of adaptability. It can evolve with modern paradigms, empowering developers to stay updated. Despite its seemingly simple interface, React is a robust library that can be customised and expanded to cater to your unique needs, inspiring endless possibilities.
Learning it is a challenge but a journey you don't have to take alone. The community is a supportive and knowledgeable resource, always ready to help you grow and discover. While keeping up with all the updates and advancements can be overwhelming, the community ensures you're never alone in this process. I highly recommend exploring the official documentation, which provides deeper insights and visual explanations based on real code.
React is not just a tool for component-based architecture and reusability. It's a tool that can unlock a world of innovation and creativity when understood at its foundational principles. This is the sweet spot between familiarity and innovation, and from where you start your journey towards becoming an outstanding React developer.
The very beginning
#
When learning new tools to build user interfaces, you typically begin with a basic example that displays a message on the screen, such as the popular "Hello World" program. However, this approach assumes that you are already familiar with HTML, particularly how to manipulate the Document Object Model (DOM). What is the DOM? Here is documentation you can refer to dig deeper into the topic.
TLDR; the DOM is an object your browser creates after processing HTML code. You can use and modify this object to add, remove, or update elements and make possible interactions like clicking a button or expanding a list of options.
Let's start writing some code to show a message in the browser using pure JavaScript:
1<html>
2 <body>
3 <script type="module">
4 //create a div element with id="root"
5 let rootElement = document.createElement('div')
6 rootElement.setAttribute('id', 'root')
7
8 //append the created div to the body tag in the DOM
9 document.body.append(rootElement)
10
11 //now the DOM looks like this
12 //<html>
13 // <body>
14 // <div id="root" />
15 // </body>
16 //</html>
17
18 //create a second div containing the "Hello there" content
19 let element = document.createElement('div')
20 element.textContent = 'Hello there'
21
22 //append the message div to the root div
23 rootElement.append(element)
24
25 //finally the DOM looks like follows
26 //<html>
27 // <body>
28 // <div id="root">
29 // <div>Hello there</div>
30 // </div>
31 // </body>
32 //</html>
33 </script>
34 </body>
35</html>
When working with pure JavaScript code, we use the document
variable, which represents our page's whole DOM. Lines 5 and 6 tell the DOM to create a new <div>
HTML tag with id='root'
by calling the createElement
method. In line 9, we can attach this new tag to the <body>
tag by executing the append
function. Then, the code follows the same approach to create a second <div>
that will be nested to the root one, containing the text we can see on the screen.
If you feel the need to touch and tweak this code, you can experiment with it in this sandbox. Notice that all the functions we're using are from JS, so there's no need for imports or additional installations. It's just a clear, vanilla JavaScript playground.
Also, here is ReactDOM's complete API to experiment further and play with more functions.
Jumping into React
#
Learning to manipulate the DOM using pure JavaScript is important because React uses these same APIs, such as createElement
and append
, behind the scenes. Below is how the components are created and rendered on the screen but this time using the React library:
1<html>
2 <body>
3 <script type="module">
4 //get the div with id="root" from the current DOM object
5 const rootElement = document.getElementById('root')
6
7 //create a div element using React
8 let element = React.createElement('div',
9 {children: 'Hello there'}
10 )
11
12 //render the div created into the root div obtained at the beginning
13 ReactDOM.render(element, rootElement)
14 </script>
15
16 <div id="root"></div>
17 </body>
18</html>
The createElement
function might look familiar if compared with the JavaScript code. Instead of using the document
variable we use React
to execute the create function, which requires three parameters to work:
type
is the HTML tag name or the Component name ('div', 'MyFirstComponent')props
is a JavaScript object that contains all properties of the elements, such as style configurations, id, etcchildren
is a JavaScript object containing all children's elements for the element we're creating. It can be just text or a group of components
In React, the JavaScript functions are wrapped to abstract the details of how things are done. Instead, it promotes a declarative approach where we just need to provide the parameters of what we want and let React handle the execution. It's like telling a story - we don't need to define every step but rather describe what we want to happen.
Introducing ReactDOM
#
In the earlier example, you might have noticed that we used React
and ReactDOM
. React
creates elements, while ReactDOM
places them in the DOM. As soon as we request an element using React
, ReactDOM
jumps in and puts it in the right spot using the render
method.
Let's look at a tougher example. Keep an eye on the props
and children
parameters. You can play around with it in the sandbox if you want to:
1<html>
2 <body>
3 <script type="module">
4 //get the div tag with id="root"
5 const rootElement = document.getElementById('root')
6
7 //use React to create the element for the "Hello" text
8 const textElement = React.createElement('span', {
9 key: 'first',
10 style: { color: 'blue' },
11 children: 'Hello',
12 })
13
14 //globe SVG icon
15 const path = React.createElement('path', {...});
16
17 //use React create an element to contain the SVG icon
18 const svgElement = React.createElement('svg', {
19 className: "svg",
20 viewBox: "0 0 24 24",
21 width:"24",
22 height:"24",
23 key: 'second',
24 },
25 path
26 )
27
28 //define the parameters for the createElement function so it creates a div
29 //to contain the elements and its className parameter as 'appContainer'
30 const elementType = 'div'
31 const elementProps = {
32 className: 'appContainer',
33 }
34 const children = [textElement, ' ', svgElement]
35
36 //use React to create the container
37 const element = React.createElement(elementType,
38 elementProps,
39 children
40 )
41
42 //use ReactDOM to locate the element in the root div
43 ReactDOM.render(element, rootElement)
44 </script>
45
46 <div id="root"></div>
47 </body>
48</html>
Please take a moment to check out when the text element is created (lines 8 to 12). The children
property is part of the props
object. However, we can also opt for using the third parameter of the create function as in the svgElement
(lines 18 to 26). This feature is useful as it prevents us from writing additional code when spreading the component's properties. We will make it there, promise!
Deep Dive
Using SVG images on your website is an excellent way to define shapes or backgrounds that retain their quality no matter how large the client's screen is. If you're curious and want to learn more about building these images, I recommend watching this video.
Summary
#
Today we discussed some basics and drew the line between what we know and what's new. Then, we looked at React's top-level API and discovered that it executes JavaScript code in the background!
By checking out both approaches, we can see that React has some cool new features that improve our experience with the tool through its declarative API. Finally, with the arrival of ReactDOM, we now have another foundation for creating HTML elements and putting them in the right place.
The Extra Mile
#
Now that you have a general taste of how React works at a basic level and how to place elements on the screen, would you like to put what we learned into practice?
Using the createElement
and append
functions from React
and ReactDOM
, try to show a list of whatever you like on the screen. You can make it as complex as you want with nested elements for each list item, adding some visual effects, which can be a rewarding challenge. However, a simple unordered list (<ul>
) with a couple of list items (<li>
) inside would also do the trick. Here is my solution in case it helps π
In the next post, we will create components the way you will from now on. We will also discuss how the browser interprets the code we return from the component and converts it into HTML. Finally, we will review some interesting new concepts and features that build on the foundations we learned today.
Looking forward to seeing you then!